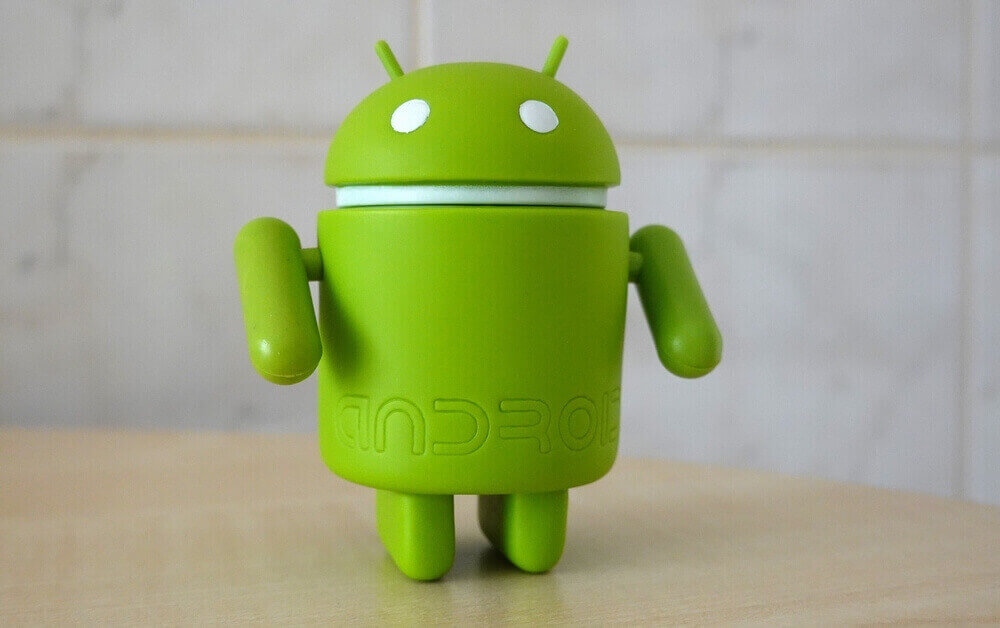
2 ways to copy directory recursively in Kotlin.
This article explains how to do it and gives code examples
1. Use Files.copy() method
First way is using Files.copy() method.
Sample code in Kotlin :
/// Copy source directory val srcDir: File = ... /// Copy destination directory val destDir: File = ... /// Copy src to dest Files.copy(destDir.toPath(), FileOutputStream(targetDir))
3 ways to call Files.copy() :
static fun copy(
source: Path!,
target: Path!,
vararg options: CopyOption!
): Path!static fun copy(
in: InputStream!,
target: Path!,
vararg options: CopyOption!
): Longstatic fun copy(
source: Path!,
out: OutputStream!
): LongQuotes : https://developer.android.com/reference/kotlin/java/nio/file/Files#copy
Looks so simple and good way.
But you can use this method only API level 26 or more.
2. Use File.copyRecursively() method
This way is more useful to copy directory recursively.
Summary of this method :
fun File.copyRecursively(
target: File,
overwrite: Boolean = false,
onError: (File, IOException) -> OnErrorAction = { _, exception -> throw exception }
): BooleanCopies this file with all its children to the specified destination target path. If some directories on the way to the destination are missing, then they will be created.If this file path points to a single file, then it will be copied to a file with the path target. If this file path points to a directory, then its children will be copied to a directory with the path target.If the target already exists, it will be deleted before copying when the overwrite parameter permits so.
Quotes : https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.io/java.io.-file/copy-recursively.html
In Kotlin, this method can be used regardless of the API level.
Sample code in Kotlin :
/// Copy source directory val srcDir: File = ... /// Copy destination directory val destDir: File = ... /// Copy directory recursively val overwrite = true srcDir.copyRecursively(destDir, overwrite){ file, e -> System.out.println("Failed to copy : ${e.message}") /// SKIP or TERMINATE OnErrorAction.SKIP }
Error handling is supported.
Related articles…
These articles may may also help you 🙂
👇 How to zip directories & files on Android using Kotlin