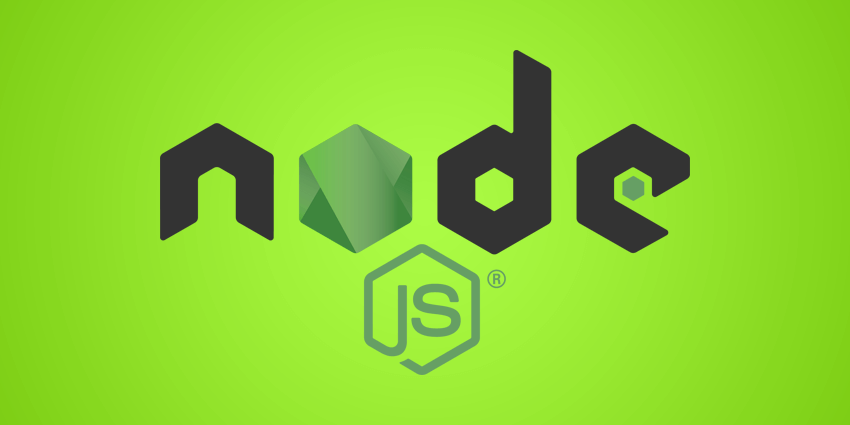
How to get browser languages in Node.js (Express.js).
This article explains 2 ways to do so with example codes.
What’s Accept-Language header?
You can get browser languages by Accept-Language header.
What’s Accept-Language header? :
The Accept-Language request HTTP header indicates the natural language and locale that the client prefers. The server uses content negotiation to select one of the proposals and informs the client of the choice with the Content-Language response header. Browsers set required values for this header according to their active user interface language. Users rarely change it, and such changes are not recommended because they may lead to fingerprinting.
Quote : https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Accept-Language
Example of Accept-Language header :
fr-CH, fr;q=0.9, en;q=0.8, de;q=0.7, *;q=0.5
This Accept-Language header contains a comma-separated list of 2- to 3-character language codes and optional subtags that the browser client can recognize.
To obtain the language codes, simply parse this header.
Get language codes by using regex
First way is parsing Accept-Language header by regex.
Sample code to parse language codes :
const acceptLanguage = 'fr-CH,fr;q=0.9,en;q=0.8,de;q=0.7,*;q=0.5'; const langs = acceptLanguage.split(',') .reduce((arr, lc)=>{ let [code, q] = lc.split(';'); if(q == undefined){ q = 'q=1.0' } arr.push({code, q:q.split('=')[1]}) return arr; }, []); console.log('langs : ', langs);
The Output of the above code :
langs : [ {code: 'fr-CH', q: '1.0'}, {code: 'fr', q: '0.9'}, {code: 'en', q: '0.8'}, {code: 'de', q: '0.7'}, {code: '*', q: '0.5'} ]
Sample code in Node.js :
const http = require("http"); http.createServer(function(req, res) { const acceptLanguage = req.headers["accept-language"]; const langs = acceptLanguage.split(',').reduce((arr, lc)=>{ let [code, q] = lc.split(';'); if(q == undefined){ q = 'q=1.0' } arr.push({code, q:q.split('=')[1]}) return arr; }, []); console.log('langs : ', langs) /// bla bla bla... }).listen(3000)
Accept-Languge is parsed as an object array with language codes and weights.
Get languages by locale library
Second way is using locale package which is Node.js library.
Github – florrain / locale :
https://github.com/florrain/locale
Install locale package :
$ npm install locale
Sample code to use locale in Node.js + Express.js :
const http = require("http"); const express = require("express"); const locale = require("locale"); const supported = ["en", "en_US", "ja"]; const default = "en"; const app = express(); app.use(locale(supported, default)) app.get("/", function(req, res) { res.header("Content-Type", "text/plain"); res.send( "You asked for: " + req.headers["accept-language"] + "\n" + "We support: " + supported + "\n" + "Our default is: " + locale.Locale["default"] + "\n" + "The best match is: " + req.locale + "\n" ) }); app.listen(3000);
Too simple and easy to use!!
Related articles
Below articles may also be useful.
Bye 🙂