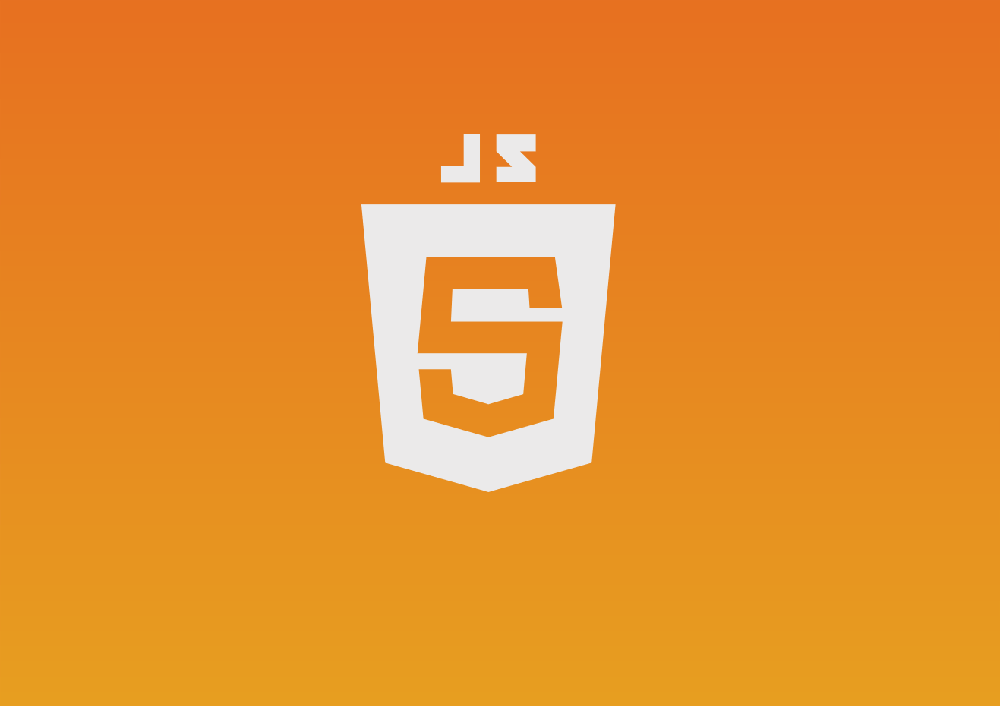
How to get a cookie value by key / name in javaScript.
This article explains the three methods with sample code.
1. Get a cookie by split() and find()
The first method is the steady way.
document.cookie returns a semi-colon delimited string.
Try showing all cookie :
console.log(document.cookie);
This code output :
'_ga=GA1.2.951224888.1888960510; preferredlocale=en; _gid=GA1.2.1711190973.3673668910; test1=1234; test2=5678; '
Each cookie is represented in the format “key=value” and they are separated by a semicolon (;). This format remains the same regardless of the browser or environment.
So you can get the cookie value from the key in the following way.
Sample code to get a cookie by name :
/// Set a cookie document.cookie = 'test=1234;max-age=86400;path=/'; /// Get a cookie value for name 'test' const value = document.cookie .split(/;\s*/).find(row => { [key,value] = row.split('=') return key === 'test' }).split('=')[1]; console.log(value); /// => '1234'
it’s a reliable method.
2. Get a cookie by using regex
You can get a cookie by using regular expression.
Sample code to get a cookie by key :
/// Set a cookie document.cookie = 'test=1234;max-age=86400;path=/'; try{ const key = 'test'; const value = document.cookie.match( new RegExp(key+'\=([^\;]*)\;*') )[1]; console.log(value); /// => '1234' }catch(err){ console.error('Not found : ', err); }
Uses the following regular expression.
new RegExp(key+'\=([^\;]*)\;*')
We use RegExp objects rather than regular expression literals because we need to include variables. The regular expression was assembled considering that the last cookie in document.cookie is not followed by a semicolon (;).
3. Using library like js-cookie
The last one is the ultimately easy way.
Just use a library like js-cookie :
https://github.com/js-cookie/js-cookie
You can get a cookie value by so simple way.
Sample code to get cookie by js-cookie :
Cookies.get('name') // => 'value' Cookies.get('nothing') // => undefined
So simple and easy to use 🙂
If you don’t mind installing a library, try this library.