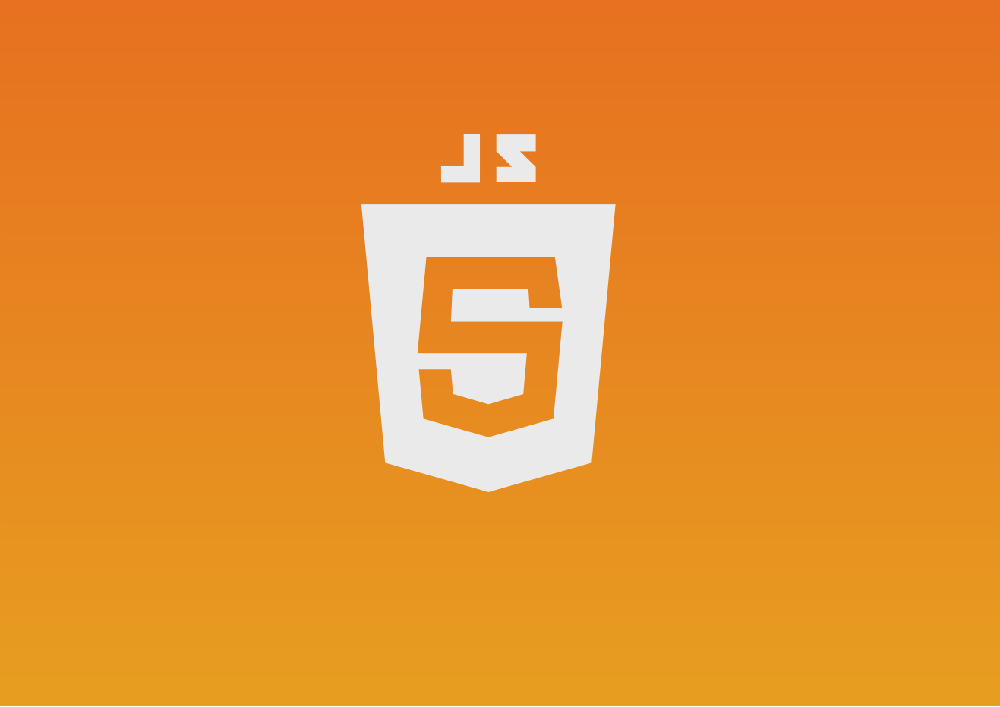
How to get Object keys as string in JavaScript.
There are 3 ways to do that.
This article explains the 3 ways with sample codes.
1. Use Object.keys() method
First way is using Object.keys() method.
It returns a key array of objects.
Example code to use Object.keys() :
const planToIdMap = { LITE: "123", BASIC: "231", PRO: "312" }; console.log( Object.keys(planToIdMap) );
Output result of the above code :
[ 'LITE', 'BASIC', 'PRO' ]
This method is often used to retrieve all key names of an object in JavaScript. However, for nested objects with more than one level, the returned key array does not contain keys of two levels or more.
2. Use Object.getOwnPropertyNames()
Second way is to use Object.getOwnPropertyNames() method.
This method is similar to Object.keys().
Example code to use getOwnPropertyNames() :
const planToIdMap = { LITE: "123", BASIC: "231", PRO: "312" }; console.log( Object.getOwnPropertyNames(planToIdMap) );
Output result of the above code :
[ 'LITE', 'BASIC', 'PRO' ]
This code example returned exactly the same key array as Object.keys().
Difference from Object.keys()
Object.getOwnPropertyNames() vs Object.keys()
What are the differences between the two method?
One thread on StackOverflow answered to this question :
There is a little difference. Object.getOwnPropertyNames(a) returns all own properties of the object a. Object.keys(a) returns all enumerable own properties. It means that if you define your object properties without making some of them enumerable: false these two methods will give you the same result.
It’s easy to test:
var a = {}; Object.defineProperties(a, { one: {enumerable: true, value: 1}, two: {enumerable: false, value: 2}, }); Object.keys(a); // ["one"] Object.getOwnPropertyNames(a); // ["one", "two"]If you define a property without providing property attributes descriptor (meaning you don’t use Object.defineProperties), for example:
a.test = 21;then such property becomes an enumerable automatically and both methods produce the same array.
Quote : https://stackoverflow.com/questions/22658488/object-getownpropertynames-vs-object-keys#22658584
Either can be used for general objects.
3. Use Object.entries() method
This method returns an array of a given object’s key-value pairs.
Example code to use Object.entries() :
const planToIdMap = { LITE: "123", BASIC: "231", PRO: "312" }; const planId = "231"; /// Find plan name having ID "231" let planName = ""; Object.entries(planToIdMap).forEach((entry)=>{ const [k, v] = entry; if(v === planId){ planName = k; } }); console.log(planName) /// => BASIC
This is useful, for the above example, to find a key with a specific value in an object and assign the key name itself to a variable.