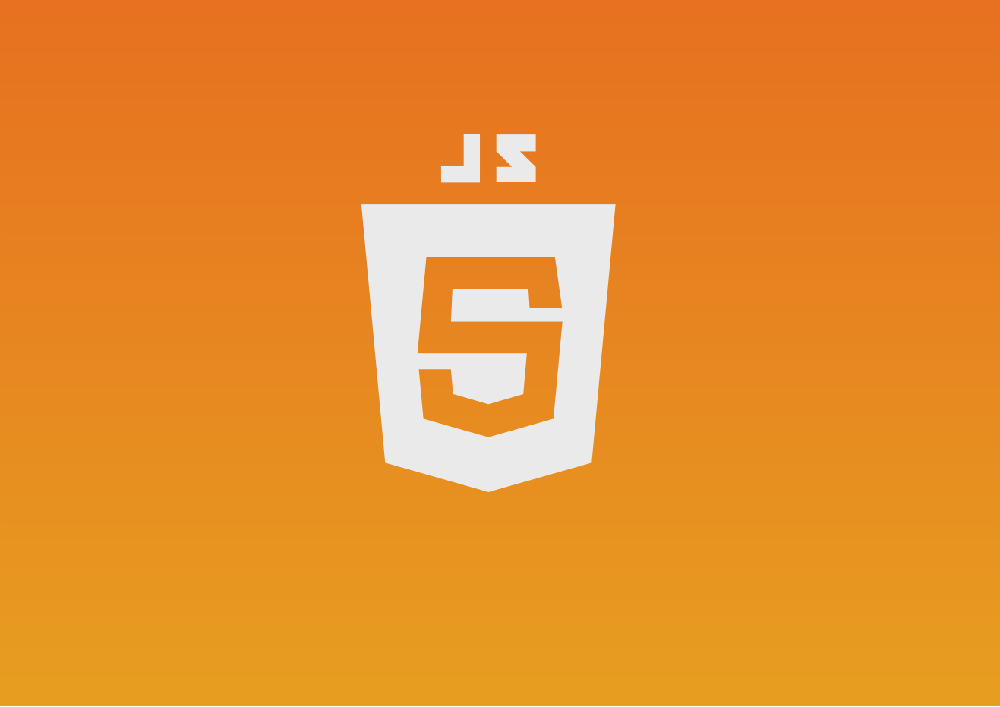
How to format Date in JavaScript.
There are 4 ways to format date time.
This article explains those methods with code examples.
1. Format by using Date.toISOString()
The first method is formatting by Date.toISOString().
What’s Date.toISOString() ? :
The toISOString() method returns a string in simplified extended ISO format (ISO 8601), which is always 24 or 27 characters long (YYYY-MM-DDTHH:mm:ss.sssZ or ±YYYYYY-MM-DDTHH:mm:ss.sssZ, respectively). The timezone is always zero UTC offset, as denoted by the suffix Z.
Quote : https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date/toISOString
Example code to format date by using this method :
const date1 = new Date(); console.log(date1.toISOString()); /// => 2023-02-21T01:47:27.185Z const date2 = new Date('2023-01-01'); console.log(date2.toISOString()); /// => 2023-01-01T00:00:00.000Z
The timestamp returned is a string representing the given date in ISO 8601 format, based on Coordinated Universal Time. ( Reference : ISO 8601 on Wikipedia)
2. Format by template literal
If you want to format the date and time on your own…
Try to use template literal.
Example code to format datetime by template literal :
/** * Utitily func to format date time. **/ function getTimeStamp(date){ return ` ${date.getFullYear()}-${date.getMonth()+1}-${date.getDate()} ${date.getHours()}:${date.getMinutes()}:${date.getSeconds()} `.trim().replace(/\s+/, ' '); } const date1 = new Date(); console.log(getTimeStamp(date1)); /// => 2023-2-21 10:58:59 const date2 = new Date('2023-01-01'); console.log(getTimeStamp(date2)); /// => 2023-1-1 9:0:0
Variables can be expanded in template literals with the statement like ${blabla} .
This is a useful approach when existing methods are insufficient.
3. Format by Date utility methods
Date also has many formatting methods not only toISOString().
List of methods that can format Date :
- Date.prototype.toDateString()
- Date.prototype.toJSON()
- Date.prototype.toLocaleString()
- Date.prototype.toLocaleDateString()
- Date.prototype.toLocaleTimeString()
- Date.prototype.toString()
- Date.prototype.toTimeString()
- Date.prototype.toUTCString()
Use them for different purposes.
4. Format by Date.js library
Date.js is useful library for parsing and formatting Date.
https://github.com/datejs/Datejs
Sample code to format date by Date.js :
new Date().toString() // "Wed Oct 31 2007 16:18:10 GMT-0700 (Pacfic Daylight Time)" new Date().toString("M/d/yyyy") // "10/31/2007" Date.today().toString("d-MMM-yyyy") // "31-Oct-2007" new Date().toString("HH:mm") // "16:18" Date.today().toString("MMMM dS, yyyy") // "April 12th, 2008"
Format Specifiers can be used for date formatting.
So useful 🙂