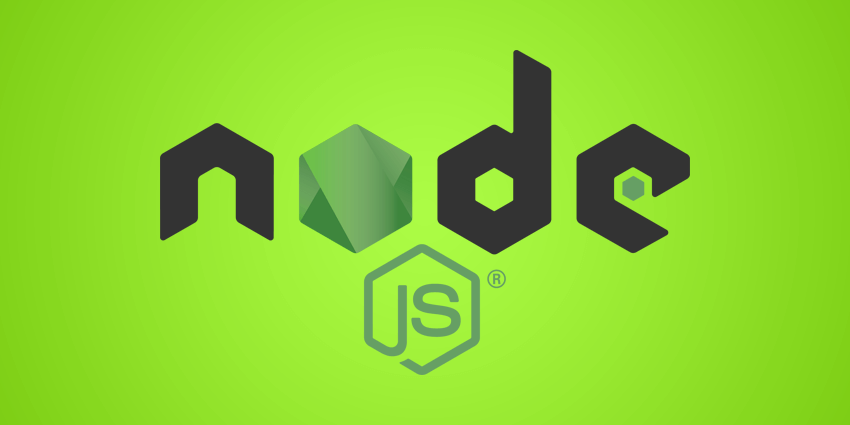
How to get country code in Node.js?
E.G : ‘US’ , ‘BR’ , ‘JP’ , ..
This article explains the way with sample codes.
1. Install request-ip module
First of all, install request-ip module.
This library can obtain user’s IP address.
Install via npm :
$ npm install request-ip
It supports both IPv4 and IPv6.
2. Install geoip-lite module
There are the library for GeoIP.
Github :
https://www.npmjs.com/package/geoip-lite
Install by npm :
$ npm install geoip-lite
This library can obtain the following country information from IP addresses.
- 2 letter country code
- 3 letter region code
- Whether in the EU or not
- Timezone
- City name
- Latitude, longitude
- Metro code
It’s not only the country code that can be obtained.
3. Get country code by geoip-lite
Now let’s get country code by geoip-lite.
Sample code in Node.js + Express :
const geoip = require('geoip-lite'); const requestIp = require('request-ip'); app.use(function(req, res, next) { const clientIp = requestIp.getClientIp(req); const geo = geoip.lookup(clientIp); console.log('geo : ', geo); next(); });
The output of above code :
{ range: [ 3479298048, 3479300095 ], country: 'US', region: 'TX', eu: '0', timezone: 'America/Chicago', city: 'San Antonio', ll: [ 29.4969, -98.4032 ], metro: 641, area: 1000 }
You can obtain not only country code (E.G : ‘US’ , ‘BR’, ‘JP’) but also region code (E.G : ‘TX’ = Texas), 1 or 0 indicating whether the country is in the EU or not, timezone, city name, latitude and longitude etc…
If only country code is needed…
You do not need geoip-lite if you only want to get the country code.
You can use the geoip-country module instead.
Github : geoip-country
https://www.npmjs.com/package/geoip-country
Install geoip-country via npm
$ npm install geoip-country
Sample code to get country code :
const geoip = require('geoip-country'); const requestIp = require('request-ip'); app.use(function(req, res, next) { const clientIp = requestIp.getClientIp(req); const geo = geoip.lookup(clientIp); console.log('geo : ', geo); next(); });
The output of the above code :
{ range: [ 3479299040, 3479299071 ], country: 'US'}
The unpacked size of geoip-lite is 115 MB, too huge just to get the country code. On the other hand, geoio-country is lightweight at 11.4MB. I recommend using this one.
Related articles
This article may be useful.
Good luck 👍