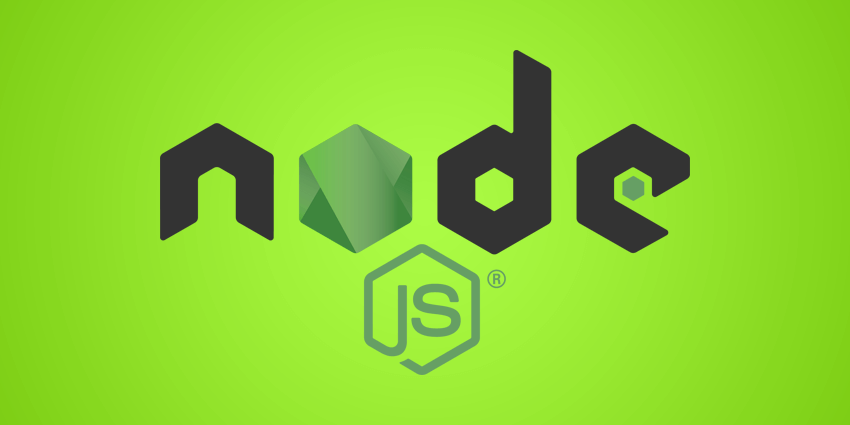
How to generate random UUID v4 in Nodejs.
There are 3 ways to do that.
This article shows the 3 ways with code examples.
1. Use built-in crypto module
The first way is to use the built-in module crypto.
NOTE : Node.js 14.17.0 or later only
Sample code to generate UUID v4 in node.js :
const crypto = require("crypto"); const uuid = crypto.randomUUID() console.log(uuid); // => 5db856b3-197b-4101-8b8d-9f43a60cef4c
Quote : crypto.randomUUID() :
Generates a random RFC 4122 version 4 UUID. The UUID is generated using a cryptographic pseudorandom number generator.
https://nodejs.org/docs/latest-v14.x/api/crypto.html#crypto_crypto_randomuuid_options
Note : Only works with Node.js 14.17.0 or later.
If you are using older Node.js than that, try the following alternatives.
2. Install UUID module
The second way is to use UUID module.
Most recommended module is uuidjs/uuid library.
Github : https://github.com/uuidjs/uuid
Install uuid module via npm :
$ npm install uuid
Sample code to generate random UUID v4 :
import { v4 as uuidv4 } from 'uuid'; const uuid = uuidv4(); console.log(uuid); /// => 84b0ad70-e320-4c45-935e-4333ba062489
So easy to use.
In addition, this module supports UUID v1 through v3.
3. Create UUID utility method
The last way is to create UUID method by your own.
Sample code to create UUID method in Node.js :
const uuidv4 = () => { return ([1e7]+-1e3+-4e3+-8e3+-1e11).replace(/[018]/g,c => (c^(crypto.randomBytes(1)[0]&(15>>(c/4)))).toString(16) ); }; const uuid = uuidv4(); console.log(uuid); /// => 74f7c0bf-abbe-4ce1-b1cc-98ac43a9d9c1
Whether it is practical or not has not been tested.
Related articles
The articles below may be helpful.
Make fixed length random number / string in JavaScript with sample codes
Bye 🙂