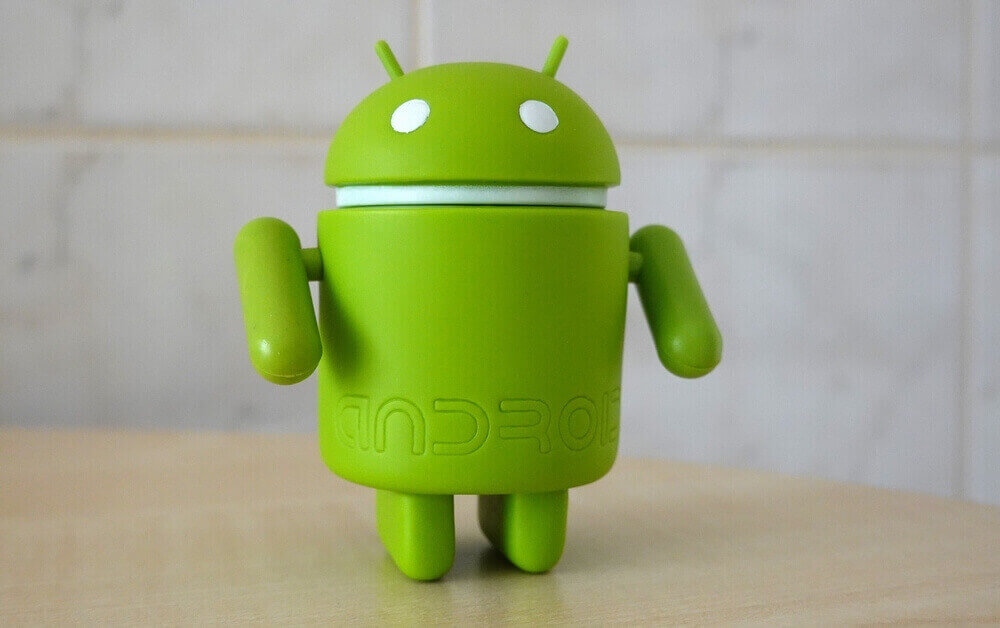
How to display AdMob interstitial ad before opening next activity.
- Initialize & load interstitial ad
- Show interstitial before next activity
- Wait till the user close the ad
- Open next activity
This article explains the procedure in Android & Kotlin.
1. Initialize interstitial ad
First of all, Initialize interstitial ad in your activity.
Sample code : define unit ID & InterstitialAd
class MainActivity: AppCompatActivity(){ /// AdMob unit ID val ADMOB_UNIT_ID_INTERSTITIAL = "ca-app-pub-1234567890123456/1234567890" /// InterstitialAd var interstitialAd: InterstitialAd? = null ... }
Sample code : The method to Initialize and load InterstitialAd
fun loadInterstitialAd() { InterstitialAd.load( this, ADMOB_UNIT_ID_INTERSTITIAL, AdRequest.Builder().build(), object : InterstitialAdLoadCallback() { override fun onAdLoaded(interstitialAd: InterstitialAd) { super.onAdLoaded(interstitialAd) this@MainActivity.interstitialAd = interstitialAd } override fun onAdFailedToLoad(loadAdError: LoadAdError) { super.onAdFailedToLoad(loadAdError) this@MainActivity.interstitialAd = null } } ) }
This code is valid for AdMob SDK ver20 or later.
If you are using an older version, please update to the latest AdMob SDK. (Related AdMob official post – Deprecation and sunset : https://developers.google.com/admob/android/deprecation)
2. Create method to show interstitial
Suppose you want to open the next Activity after displaying interstitial ad.
For this purpose, we have prepared the following method
Sample code : method to show interstitial
fun showInterstitialAd(onAdFinished: () -> Unit){ if(interstitialAd != null){ interstitialAd?.show(this) interstitialAd?.fullScreenContentCallback = object : FullScreenContentCallback(){ override fun onAdDismissedFullScreenContent() { onAdFinished() } override fun onAdShowedFullScreenContent() { interstitialAd = null loadInterstitialAd() } } }else{ onAdFinished() loadInterstitialAd() } }
This method takes a callback function named onAdFinished as an argument to pass the processing to be performed after the ad is displayed.
If the interstitial ad is loading, the callback is executed after the ad is finished displaying; if it is loading, the callback is executed immediately.
3. Show interstitial before next activity
Use the method defined above
Sample code :
showInterstitialAd { val intent = Intent(this@MainActivity, NextActivity::class.java) startActivity(intent) }
After the ad is displayed and the user closes it, NextActivity is launched.
This is the best way to display interstitials in AdMob because the destination Activity has no performance impact on ad display.
Good post. thx 🙂