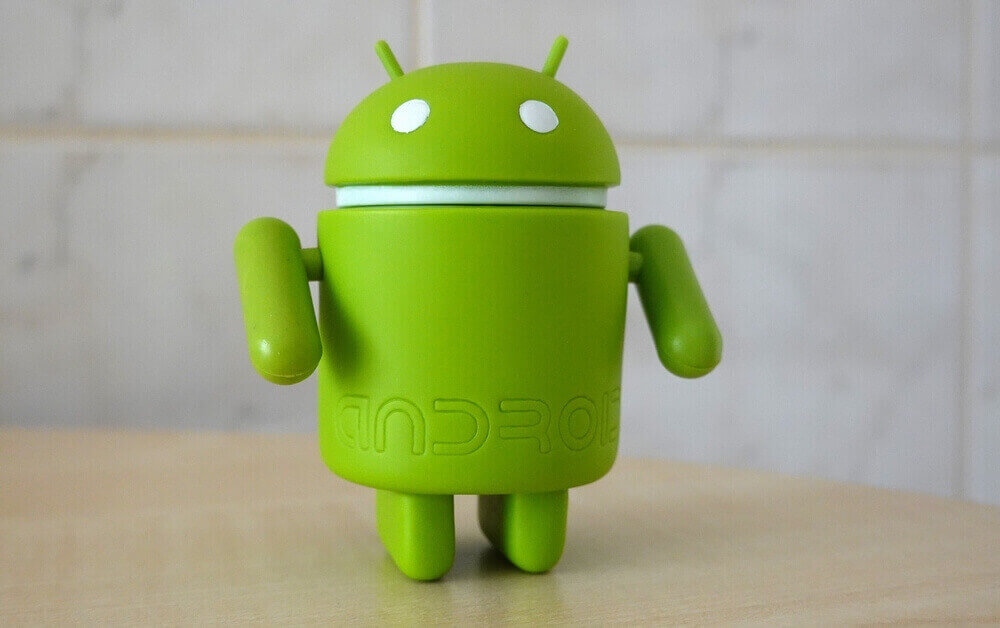
ProgressDialog has been deprecated in Android since API level 26.
Quote : ProgressDialog | Android developer
This class was deprecated in API level 26.
ProgressDialog
is a modal dialog, which prevents the user from interacting with the app. Instead of using this class, you should use a progress indicator like ProgressBar, which can be embedded in your app’s UI. Alternatively, you can use a notification to inform the user of the task’s progress.URL : https://developer.android.com/reference/android/app/ProgressDialog
So you need to display ProgressDialog in your own implementation.
The alternative methods are presented in this article.
1. Create layout for ProgressDialog
Create custom layout for ProgressDialog.
Sample code – dialog_progress.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent"> <LinearLayout android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginStart="16dp" android:layout_marginTop="16dp" android:layout_marginEnd="16dp" android:layout_marginBottom="16dp" android:orientation="vertical" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent"> <ProgressBar android:id="@+id/progress_bar" style="@style/Widget.AppCompat.ProgressBar.Horizontal" android:layout_width="match_parent" android:layout_height="wrap_content" /> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="horizontal"> <TextView android:id="@+id/progress_1_text_view" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:text="0%" /> <TextView android:id="@+id/progress_2_text_view" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:gravity="right" android:text="0/100" /> </LinearLayout> </LinearLayout> </androidx.constraintlayout.widget.ConstraintLayout>
The layout having 1 ProgressBar and 2 TextView
2. Create a class extending DialogFragment
Create ProgressDialog class extemding DialogFragment
Sample code – ProgressDialog.kt
class ProgressDialog : DialogFragment() { private lateinit var progressBar: ProgressBar private lateinit var progress1TextView: TextView private lateinit var progress2TextView: TextView companion object { fun newInstance(title: String, message: String): ProgressDialog { val instance = ProgressDialog() val arguments = Bundle() arguments.putString("message", message) arguments.putString("title", title) instance.arguments = arguments return instance } } override fun onCreateDialog(savedInstanceState: Bundle?): Dialog { val title = requireArguments().getString("title") val message = requireArguments().getString("message") val builder = AlertDialog.Builder(requireActivity()) val inflater = requireActivity().layoutInflater val view = inflater.inflate(R.layout.dialog_progress, null) progressBar = view.findViewById(R.id.progress_bar) progress1TextView = view.findViewById(R.id.progress_1_text_view) progress2TextView = view.findViewById(R.id.progress_2_text_view) builder.setTitle(title) builder.setMessage(message) builder.setView(view) return builder.create() } fun setProgress(progress: Int){ if(this::progressBar.isInitialized) { progressBar.progress = progress progress1TextView.text = "${progress}%" progress2TextView.text = "${progress}/100" } } }
This class has minimal functionality as a ProgressDialog.
- Show title & message
- Show & update progress on ProgressBar
- Show & update progress text
Customize this class as needed.
3. Show & update ProgressDialog
Finally you can show ProgressDialog.
Sample code in MainActivity.kt
/// Show ProgressDialog with title & msg val progressDialog = ProgressDialog.newInstance( "Progress Dialog", "Downloading...") progressDialog.show(supportFragmentManager, TAG) /// Simulation of download process progressDialog.setprogress(10) ... progressDialog.setprogress(50) ... progressDialog.setprogress(100)
Example of displayed progressDialog (51/100) :
Example of displayed ProgressDialog (100/100)
Well done!!
Wow that was strange. I just wrote an extremely long comment but after I clicked submit my comment didn’t show up. Grrrr… well I’m not writing all that over again. Anyways, just wanted to say excellent blog!
Greetings from Florida! I’m bored to death at work so I decided to check out your site on my iphone during lunch break. I love the information you provide here and can’t wait to take a look when I get home. I’m surprised at how quick your blog loaded on my mobile .. I’m not even using WIFI, just 3G .. Anyways, excellent blog!
Hello there! I know this is somewhat off topic but I was wondering which blog platform are you using for this site? I’m getting tired of WordPress because I’ve had problems with hackers and I’m looking at alternatives for another platform. I would be great if you could point me in the direction of a good platform.
Attractive section of content. I simply stumbled upon your website and in accession capital to assert that I get actually enjoyed account your weblog posts. Any way I抣l be subscribing in your augment or even I success you access consistently quickly.
I need to install anti-spam plugin lol. bye