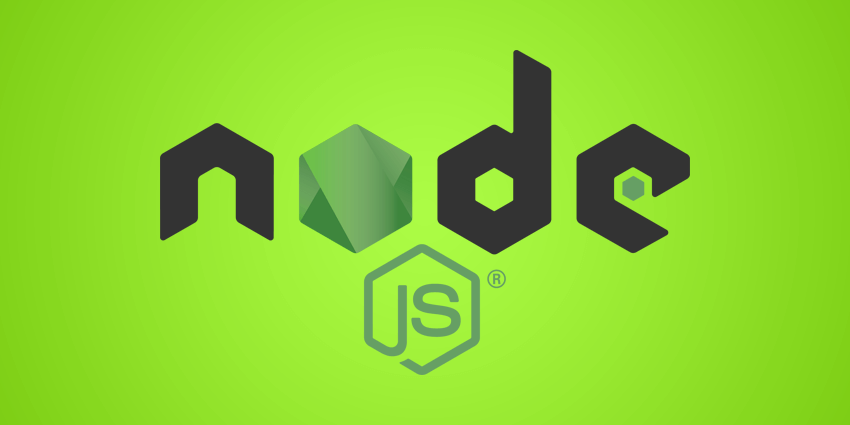
How to create password hash in Node.js.
This article explains the way and code examples.
The steps are as follows…
1. Install bcrypt library
First of all, you need to install bcrypt.
This library helps you to hash password or something.
Install via npm :
> npm install bcrypt
Latest version is 5.1.0.
If your node version is 11 or less, you must note version compatibility with bcrypt.
Bcrypt version Compatibility :
Node Version Bcrypt Version 0.4 <= 0.4 0.6, 0.8, 0.10 >= 0.5 0.11 >= 0.8 4 <= 2.1.0 8 >= 1.0.3 < 4.0.0 10, 11 >= 3 12 onwards >= 3.0.6 Quotes : https://www.npmjs.com/package/bcrypt
You had better to upgrade to v5.0.0 to avoid security issues.
2. Create password hash
You can create password hash by the below codes.
Example code to create password hash :
const bcrypt = require("bcrypt"); async function getPasswordHash(password){ return await bcrypt.hash(password, 10); }
Just use bcrypt.hash() with passing password and salt rounds.
e.g. Use this method in Express.js router… :
router.post('/create-hash', async (req, res, next) => { /// Raw password const password = req.body.password; /// Create password hash const passwordHash = await getPasswordHash(password); /// bla bla bla... });
Storing passwords in plain text in the database is to be avoided.
Storing password hashes instead improves security.
3. Compare hash and password
You can use bcrypt.compare() to match hash against raw password.
Example code to compare hash and password :
async function comparePassword(password, hash){ /// true or false return await bcrypt.compare(password, hash); }
Return true if password and hash match.
Note. Hash isn’t same for same input
Created password hash is always different for same password.
For example, if you create a hash for “My Password” …
e.g. First attempt:
$2b$10$wygSOPYzFUuxFYz.jMVcWONAtXnll.lHCPXdAOIKB2ipKkeVnJCp2
e.g. Second attempt :
$2b$10$pO7Yiy4Y8L3jnhH9La.7b.2mCJoK5WDaItXcsoiW8dRoSDRizACji
e.g. Third attempt :
$2b$10$PlY.tQ5fiTqVyDMcH2bThOqoCYl/Ru1JgHXqkI.6LhVYBKCaQLjem
The hash for the same password will be different each time.
Therefore, when comparing a password to a hash using bcrypt, it is fatally incorrect to create a new hash from that password and compare it to the original hash. :< :<
There is no other way to compare passwords and hashes except by using bcrypt.compare(password, hash).