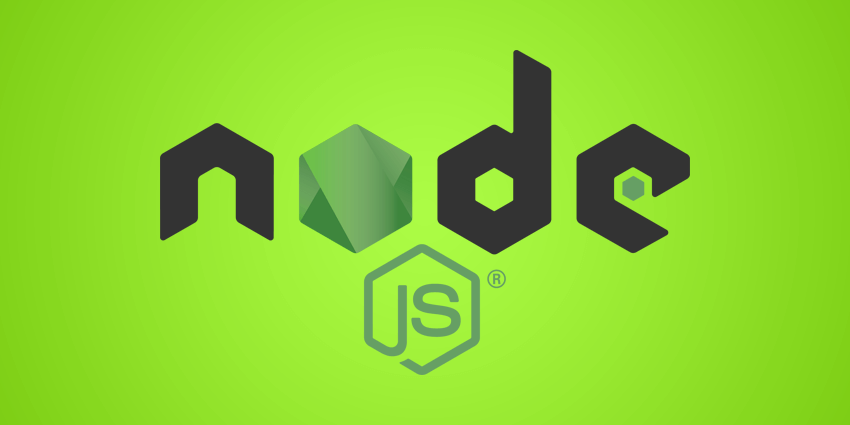
How to use shared memory cache in Node.js.
Node.js itself doesn’t have a memory cache function.
So a module for memory cache is needed.
This article explains how to use that module with example code.
Which module should you choose?
There are many modules for shared memory caching.
As far as I know, there are 3 modules for Node.js.
Which module on earth should we choose?
The best module is node-cache
The best one is [node-cache/node-cache]
Github : https://github.com/node-cache/node-cache
Why this module should be used? :
- Supports caching of values, arrays, and objects
- TTL can be specified for cache
- Maximum number of caches can be specified
- Can watch current memory consumption
- Simple and fast caching
Its arguably the best library for shared memory caching.
Install node-cache module via npm
Let’s install node-cache module first of all.
Install it via npm :
$ npm install node-cache
Incidentally this library is MIT licensed.
How use shared memory cache
You can use node-cache for shared memory caching like this.
Example code to use memory cache in Node.js :
/// Require Node-Cache module const NodeCache = require( "node-cache" ); const nodeCache = new NodeCache(); const http = require('http'); http.createServer((req, res)=>{ /// Get / Set a value to the shared memory cache. let value = nodeCache.get('valueKey'); if(value == undefined){ /// the TTL is 3600 seconds value = 123 success = nodeCache.set( "valueKey", value, 60*60 ); } /// Get / Set an array to the shared memory cache. let arr = nodeCache.get('arrayKey'); if(arr == undefined){ arr = ['a', 'b', 'c']; success = nodeCache.set( "arrayKey", arr, 60*60 ); } /// Get / Set an object to the shared memory cache. let obj = nodeCache.get('objectKey'); if(obj == undefined){ obj = {a:1,b:2,c:3} success = nodeCache.set( "objectKey", obj, 60*60 ); } res.writeHead(200, {'Content-Type': 'text/plain'}); res.end('bla bla bla'); }).listen(8080);
If the cache already exists, NodeCache.get(key) returns the cached value. If not, undefined is returned, so use NodeCache.set(key,value,ttl) to add the value to the shared memory cache.
It’s so easy to use module.
Limit max numbers of caches
The library also allows the maximum number of caches to be specified.
Example code to set max number of caches :
/// Require Node-Cache module. /// Set 10000 to max number of caches. const NodeCache = require( "node-cache" ); const nodeCache = new NodeCache({ maxKeys: 10000 }); const http = require('http'); http.createServer((req, res)=>{ /// Get / Set a value to the shared memory cache. let value = nodeCache.get('valueKey'); try{ if(value == undefined){ value = 123 success = nodeCache.set( "valueKey", value, 60*60 ); } }catch(err){ /// If the cache limit is exceeded, reach here. value = 123; } res.writeHead(200, {'Content-Type': 'text/plain'}); res.end('bla bla bla'); }).listen(8080);
Just create NodeCache with maxKeys option.