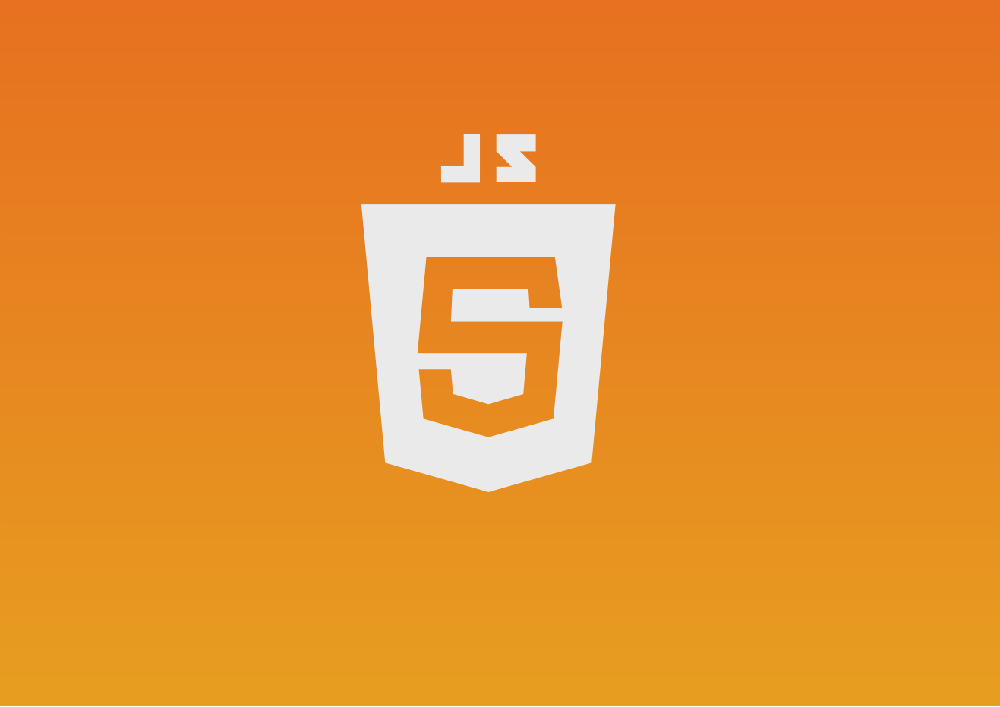
How to make the following in JavaScript…
- Fixed length random numbers,
- Fixed length random string,
This article explains how to do it in JavaScript with example codes.
Make fixed length random numbers
How to make random number with fixed length.
- Ex1 : 276199428
- Ex2 : 129498289
Create a random number generator function as follows :
function randomNumbers(length) { var result = ''; var chars = '0123456789'; var charsLength = chars.length; for ( var i = 0; i < length; i++ ) { result += chars.charAt( Math.floor(Math.random() * charsLength) ); } return result; }
Generate random numbers by using the above func :
/// Generate 5 length random numbers randomNumbers(8); /// => '13432233' randomNumbers(8); /// => '58069170' /// Generate 20 length random numbers randomNumbers(20); /// => '29678817177096512499' randomNumbers(20); /// => '80786866995930267929' randomNumbers(20); /// => '52494552172139615664'
Any-digit number can be generated.
Make fixed length string [a-zA-Z]
How to make fixed length string with fixed length.
- Ex1 : ‘TrlTgzDyDm’
- Ex2 : ‘HnFNoPMfeu’
Create a string generator function as follows :
function randomString(length) { var result = ''; var chars = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz'; var charsLength = chars.length; for ( var i = 0; i < length; i++ ) { result += chars.charAt( Math.floor(Math.random() * charsLength) ); } return result; }
Example usages of the above function :
/// Make 5 length random string randomString(5); /// => 'a2FeC' randomString(5); /// => 'we5M2' /// Make 10 length random string randomString(10); /// => 'UAZ2iKAUcm' randomString(10); /// => 'BZKQRMQW8V'
Strings containing only letters [a-zA-Z] of fixed length are generated.
Generate fixed length hex code
Random hex numbers with fixed digits can also be generated.
Create a hex code generator function :
function randomHex(length) { let result = ''; const chars = '0123456789abcdef'; const charsLength = chars.length; for ( var i = 0; i < length; i++ ) { result += chars.charAt( Math.floor(Math.random() * charsLength) ); } return result; }
Make random hex code by the above func :
/// Make 6 length hex codes randomHex(8); /// => '4ca9cd32' randomHex(8); /// => 'fd7f679f' /// Make 16 length hex codes randomHex(16); /// => 'ff7198af24f25da3' randomHex(16); /// => '0b4d19a0c7db1e04' randomHex(16); /// => '3d9b4f71456c8ea9'
Generate random binary string
Create a binary string generator function :
function randomBin(length) { let result = ''; const chars = '01'; const charsLength = chars.length; for ( var i = 0; i < length; i++ ) { result += chars.charAt( Math.floor(Math.random() * charsLength) ); } return result; }
Make random binary strings by the above func :
/// Make 8 length binary strings randomBin(8); /// => '11010100' randomBin(8); /// => '11100111' randomBin(8); /// => '00001001'
Other n-decimal systems (e.g., octal / ternary) can also be generated randomly with any number of digits by restricting the types of characters that can be included.