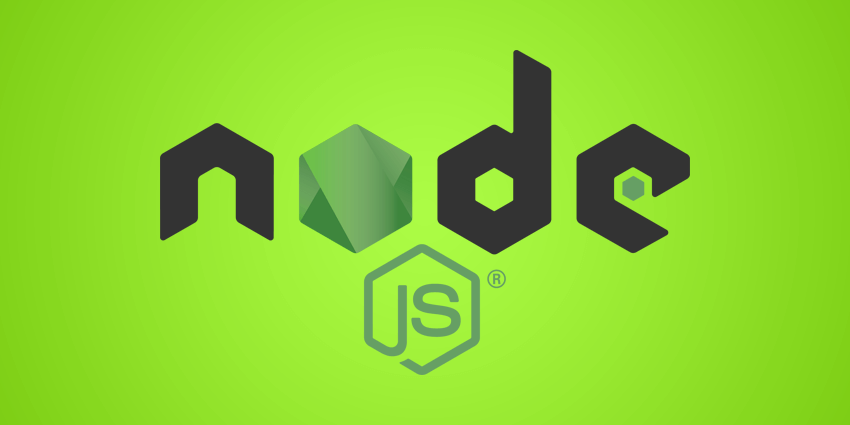
How to use regex in express router in node.js with sample codes.
This article explains how to do it with code examples.
Use regex in router path
In fact, simply pass a regular expression.
Very simple sample code :
const express = require('express'); const router = express.Router(); // Route which matches regex router.get(/YOUR REGEX/, (req, res, next) => { res.send("Hello"); }); /// Or pass regex object const regexp = new RegExp('YOUR REGEX'); router.get(regexp, (req, res, next) => { res.send("Hello"); });
Pass regex literal or RegExp object.
For more details, see the below reference.
Regular expressions are patterns used to match character combinations in strings. In JavaScript, regular expressions are also objects. These patterns are used with the exec() and test() methods of RegExp, and with the match(), matchAll(), replace(), replaceAll(), search(), and split() methods of String. This chapter describes JavaScript regular expressions.
Quotes : https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Regular_Expressions
Specific routing examples will now be presented.
Ex1. Number only router path
If you want to route a path with only numbers like the below…
- localhost:3000/20230102
- localhost:3000/134703
Try the following code :
const express = require('express'); const router = express.Router(); // Route which matches only numbers router.get(/\/[0-9]+/, (req, res, next) => { res.send("Hello"); });
Just pass a regex /[0-9]+/ representing numbers.
Ex2. Alphabet only router path
If you want to route a path with only alphabets like the below…
- localhost:3000/pikachu-is-cute
- localhost:3000/Pikachu-Is-Cute
Hyphens and underscores are also included
Sample code to do it :
const express = require('express'); const router = express.Router(); // Route which matches only alphabets router.get(/\/[a-zA-Z\-\_]+/, (req, res, next) => { res.send("Hello"); });
It works well.
Use regex in router URL params
If you want to use regular expressions for URL parameters as well…
It’s possible to embed regex directly in router path.
Sample code :
const express = require('express'); const router = express.Router(); // Use regex for name URL parameter. router.get('/archives/:date([0-9]{4}/(0[1-9]|1[0-2])/', (req, res) => { const date = req.params.date; res.send({ "date": date }) })
You can embed regular expressions by enclosing them in parentheses, such as ([0-9]{4}/(0[1-9]|1[0-2]) in this code.